封面画师:T5-茨舞(微博) 封面ID:66855568
SpringMVC
1. SpringMVC执行原理
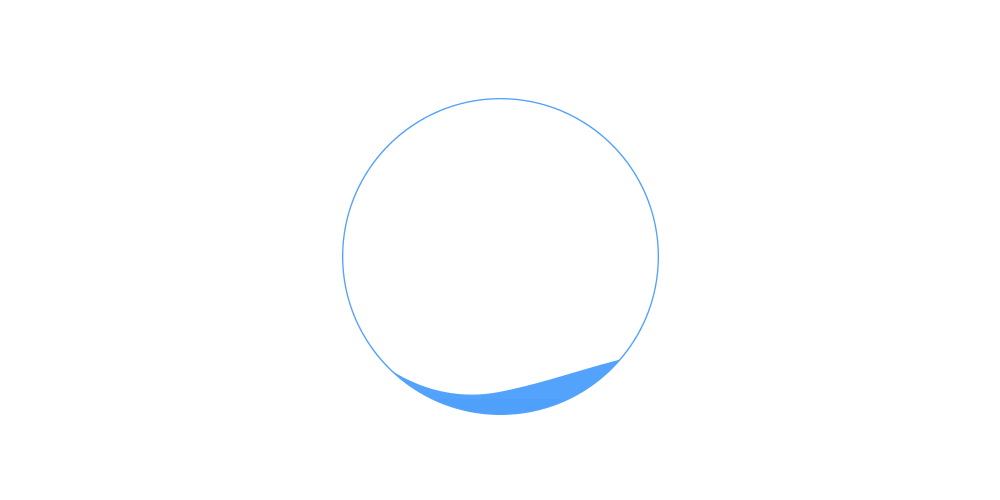
- 用户发送请求到前端控制器DispatcherServlet。
- DispatcherServlet收到请求调用HandlerMapping处理器映射器。
- 处理器映射器根据请求url(配置的bean中的name和class)找到具体的处理器,生成处理器对象及处理器拦截器(如果有则生成)一并返回给DispatcherServlet。
- DispatcherServlet通过HandlerAdapter处理器适配器调用处理器。
- 执行处理器(Controller,也叫后端控制器)。
- Controller执行完成返回ModelAndView。
- HandlerAdapter将Controller执行结果ModelAndView返回给DispatcherServlet。
- DispatcherServlet将ModelAndView传给ViewResolver视图解析器。
- ViewResolver解析后返回具体的View。
- DispatcherServlet对View进行渲染视图(即:将模型数据填充到视图中)。
- DispatcherServlet响应结果。
2. RestFul风格
2.1 功能
- 资源:互联网所有的事物都可以被抽象为资源
- 资源操作:使用POST、DELETE、PUT、GET,使用不同方法对资源进行操作。
- 分别对应 添加、 删除、修改、查询。
2.2 比较
传统方式操作资源 :通过不同的参数来实现不同的效果!方法单一,post 和 get
- /item/queryItem.action?id=1 查询,GET
- /item/saveItem.action 新增,POST
- /item/updateItem.action 更新,POST
- /item/deleteItem.action?id=1 删除,GET或POST
使用RESTful操作资源 : 可以通过不同的请求方式来实现不同的效果!如下:请求地址一样,但是功能可以不同!
- /item/1 查询,GET
- /item 新增,POST
- /item 更新,PUT
- /item/1 删除,DELETE
2.3 GET 与POST
- w3schools对于HTTP 方法:GET 和 POST
- GET后退按钮/刷新无害,POST数据会被重新提交(浏览器应该告知用户数据会被重新提交)。
- GET书签可收藏,POST为书签不可收藏。
- GET能被缓存,POST不能缓存 。
- GET编码类型application/x-www-form-url,POST编码类型encodedapplication/x-www-form-urlencoded 或 multipart/form-data。为二进制数据使用多重编码。
- GET历史参数保留在浏览器历史中。POST参数不会保存在浏览器历史中。
- GET对数据长度有限制,当发送数据时,GET 方法向 URL 添加数据;URL 的长度是受限制的(URL 的最大长度是 2048 个字符)。POST无限制。
- GET只允许 ASCII 字符。POST没有限制。也允许二进制数据。
- 与 POST 相比,GET 的安全性较差,因为所发送的数据是 URL 的一部分。在发送密码或其他敏感信息时绝不要使用 GET !POST 比 GET 更安全,因为参数不会被保存在浏览器历史或 web 服务器日志中。
- GET的数据在 URL 中对所有人都是可见的。POST的数据不会显示在 URL 中。
- GET执行效率却比POST方法好。GET是form提交的默认方法。
2.4 使用
SpringMVC中配置文件常用书写方式(springmvc-servlet.xml ):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<context:component-scan base-package="com.yang"> <context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"> </context:include-filter> </context:component-scan>
<bean id="internalResourceViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/pages/"/> <property name="suffix" value=".jsp"/> </bean>
<mvc:resources mapping="/css/**" location="/css/"/> <mvc:resources mapping="/js/**" location="/js/"/> <mvc:resources mapping="/images/**" location="/images/"/>
<mvc:annotation-driven/>
<mvc:default-servlet-handler/> </beans>
|
配置web.xml , 注册DispatcherServlet:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <servlet> <servlet-name>springmvc</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springmvc</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
|
如果使用<url-pattern>/*</url-pattern>
,会出现返回 jsp视图 时再次进入SpringMVC的DispatcherServlet 类,导致找不到对应的Controller而报404错。
RestFul风格的使用(省略界面的编写):
1 2 3 4 5 6 7 8 9
| @RequestMapping("/commit/{p1}/{p2}") public String index(@PathVariable int p1, @PathVariable String p2, Model model){ String result = p1+p2; model.addAttribute("msg", "结果:"+result); return "test"; }
|
1 2 3 4 5 6
| @RequestMapping(value = "/hello",method = {RequestMethod.GET}) public String index2(Model model){ model.addAttribute("msg", "hello!"); return "test"; }
|
2.5 小结
-
Spring MVC 的 @RequestMapping 注解能够处理 HTTP 请求的方法, 比如 GET, PUT, POST, DELETE 以及 PATCH。
-
所有的地址栏请求默认都会是 HTTP GET 类型的。提交表单则是HTTP POST 类型的。
-
方法级别的注解变体有如下几个: 组合注解
1 2 3 4 5
| @GetMapping @PostMapping @PutMapping @DeleteMapping @PatchMapping
|
@GetMapping 是一个组合注解
它所扮演的是 @RequestMapping(method =RequestMethod.GET) 的一个快捷方式。
3. 转发和重定向
3.1 区别
-
地址栏不发生变化
-
只有一个请求响应
-
可以通过request域传递数据
-
只能跳转本站点资源
-
服务器端行为
-
地址栏会发生变化
-
两次请求响应
-
无法通过request域传递对象
-
可以跳转到任意URL
-
客户端行为
3.2 SpringMVC中的使用
默认有视图解析器:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @Controller public class ResultSpringMVC { @RequestMapping("/test/t1") public String test1(){ return "test"; }
@RequestMapping("/test/t2") public String test2(){ return "redirect:/index.jsp"; } }
|
- 注意: 重定向一定不会经过视图解析器, 因为请求转发的页面是在地址栏直接访问的,是一个新的请求,如果经过视图解析器,拼接后的结果就是错误的,所以重定向一定不经过视图解析器。另外WEB-INF下的所有文件必须通过请求转发(Controller或Servlet)才能访问,重定向无法访问。
- 当用关键字(forward或redirect)实现重定向和转发时,不会使用视图解析器。
- 一般情况下,控制器方法返回字符串类型的值会被当成逻辑视图名处理。如果返回的字符串中带 forward: 或 redirect:前缀时,SpringMVC 会对他们进行特殊处理:将 forward: 和redirect: 当成指示符,其后的字符串作为 URL 来处理。( 跳页面用重定向,处理业务用转发 )
- 若控制器返回值为void,且没有经过其他特殊操作,SpringMVC会在视图解析器规定的路径下查找与@RequestMapping注解value属性值同名的界面。
4. 数据处理
4.1 处理提交数据
- 提交的域名称和处理方法的参数名一致,则可以直接接收。
- 提交的域名称和处理方法的参数名不一致:
1 2 3 4 5 6
| @RequestMapping("/hello") public String hello(@RequestParam("username") String name){ System.out.println(name); return "hello"; }
|
4.2 数据显示到前端
- 可以通过ModelAndView、ModelMap和Model三种方式。
- 区别:
1 2 3 4 5
| Model 只有寥寥几个方法只适合用于储存数据,简化了新手对于Model对象的操作和理解;
ModelMap 继承了 LinkedMap ,除了实现了自身的一些方法,同样的继承 LinkedMap 的方法和特性;
ModelAndView 可以在储存数据的同时,可以进行设置返回的逻辑视图,进行控制展示层的跳转。
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @RequestMapping("/testModelAndView") public ModelAndView testModelAndView(){ System.out.println("testModelAndView方法执行了。。。"); ModelAndView mv = new ModelAndView(); User user = new User(); user.setUsername("小王"); user.setPassword("789654"); user.setAge(12); mv.addObject("user",user);
mv.setViewName("success"); return mv; }
<a href="user/testModelAndView">testModelAndView</a><br/>
|
4.3 解决乱码问题
1 2 3 4 5 6 7 8 9 10 11 12
| <filter> <filter-name>encoding</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>utf-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>encoding</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
|
4.4 数据格式转换
- 自定义数据类型转换器。以Date数据类型为例(将输入的字符串转换成Date类型):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class StringToDateConverter implements Converter<String, Date> {
public Date convert(String source) { if(source == null){ throw new RuntimeException("请传入数据"); } DateFormat df = new SimpleDateFormat("yyyy-MM-dd"); try { return df.parse(source); } catch (Exception e) { throw new RuntimeException("数据类型转换错误"); } } }
|
1 2 3 4 5 6 7 8 9 10 11
| <bean id="connectorServerFactoryBean" class="org.springframework.context.support.ConversionServiceFactoryBean"> <property name="converters"> <set> <bean class="com.yang.utils.StringToDateConverter"/> </set> </property> </bean>
<mvc:annotation-driven conversion-service="connectorServerFactoryBean"/>
|
4.5 Servlet原生API的使用
- 只需要在控制器的方法参数定义HTTPServletRequest和HTTPServletResponse对象即可。
5. JSON
- SpringMVC中在方法上加注解 @ResponseBody 后,修饰的 方法 不会走视图解析器,只会返回一个字符串。
- SpringMVC中在类上加注解 @RestController 后,修饰的 类 下所有方法不会走视图解析器,只会返回一个字符串。
5.1 Jackson
1 2 3 4 5 6
| <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.10.0</version> </dependency>
|
简单测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Controller public class UserController { @RequestMapping("/json1") @ResponseBody public String json1() throws JsonProcessingException { ObjectMapper mapper = new ObjectMapper(); User user = new User("小明", 13, "男"); String str = mapper.writeValueAsString(user); return str; } }
|
- 使用JSON记得处理乱码问题:通过@RequestMaping的produces属性来实现,修改下代码
1 2
| @RequestMapping(value = "/json1",produces = "application/json;charset=utf-8")
|
- 还可以在springmvc的配置文件上添加一段消息StringHttpMessageConverter转换配置,实现统一指定:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <mvc:annotation-driven> <mvc:message-converters register-defaults="true"> <bean class="org.springframework.http.converter.StringHttpMessageConverter"> <constructor-arg value="UTF-8"/> </bean> <bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter"> <property name="objectMapper"> <bean class="org.springframework.http.converter.json.Jackson2ObjectMapperFactoryBean"> <property name="failOnEmptyBeans" value="false"/> </bean> </property> </bean> </mvc:message-converters> </mvc:annotation-driven>
|
输出时间对象:
Controller:
1 2 3 4 5 6 7 8 9
| @RequestMapping("/json2") public String json2() throws JsonProcessingException { ObjectMapper mapper = new ObjectMapper(); Date date = new Date(); String str = mapper.writeValueAsString(date); return str; }
|
运行结果:
-
默认日期格式会变成一个数字,是1970年1月1日到当前日期的毫秒数!
-
Jackson 默认是会把时间转成timestamps形式。
解决方案:取消timestamps形式 , 自定义时间格式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @RequestMapping("/json3") public String json3() throws JsonProcessingException { ObjectMapper mapper = new ObjectMapper(); mapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); mapper.setDateFormat(sdf);
Date date = new Date(); String str = mapper.writeValueAsString(date);
return str; }
|
抽取为工具类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| public class JsonUtils { public static String getJson(Object object) { return getJson(object,"yyyy-MM-dd HH:mm:ss"); }
public static String getJson(Object object,String dateFormat) { ObjectMapper mapper = new ObjectMapper(); mapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false); SimpleDateFormat sdf = new SimpleDateFormat(dateFormat); mapper.setDateFormat(sdf); try { return mapper.writeValueAsString(object); } catch (JsonProcessingException e) { e.printStackTrace(); } return null; } }
|
5.2 FastJson
1 2 3 4 5 6
| <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.62</version> </dependency>
|
测试类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| public class FastJsonDemo { public static void main(String[] args) { User user1 = new User("ONE", 13, "男"); User user2 = new User("TWO", 13, "男"); User user3 = new User("THREE",13, "男"); User user4 = new User("FOUR", 13, "男"); List<User> list = new ArrayList<User>(); list.add(user1); list.add(user2); list.add(user3); list.add(user4);
System.out.println("*******Java对象 转 JSON字符串*******"); String str1 = JSON.toJSONString(list); System.out.println("JSON.toJSONString(list)==>"+str1); String str2 = JSON.toJSONString(user1); System.out.println("JSON.toJSONString(user1)==>"+str2);
System.out.println("\n****** JSON字符串 转 Java对象*******"); User jp_user1=JSON.parseObject(str2,User.class); System.out.println("JSON.parseObject(str2,User.class)==>"+jp_user1);
System.out.println("\n****** Java对象 转 JSON对象 ******"); JSONObject jsonObject1 = (JSONObject) JSON.toJSON(user2); System.out.println("(JSONObject) JSON.toJSON(user2)==>"+jsonObject1.getString("name"));
System.out.println("\n****** JSON对象 转 Java对象 ******"); User to_java_user = JSON.toJavaObject(jsonObject1, User.class); System.out.println("JSON.toJavaObject(jsonObject1, User.class)==>"+to_java_user); } }
|
6. Ajax
6.1 定义
- AJAX = Asynchronous JavaScript and XML(异步的 JavaScript 和 XML)。
- AJAX 是一种在无需重新加载整个网页的情况下,能够更新部分网页的技术。
- 传统的网页(即不用ajax技术的网页),想要更新内容或者提交一个表单,都需要重新加载整个网页。使用ajax技术的网页,通过在后台服务器进行少量的数据交换,就可以实现异步局部更新。使用Ajax,用户可以创建接近本地桌面应用的直接、高可用、更丰富、更动态的Web用户界面。
6.2 jQuery.ajax
-
Ajax的核心是XMLHttpRequest对象(XHR)。XHR为向服务器发送请求和解析服务器响应提供了接口。能够以异步方式从服务器获取新数据。
-
jQuery 提供多个与 AJAX 有关的方法。
-
通过 jQuery AJAX 方法,我们能够使用 HTTP Get 和 HTTP Post 从远程服务器上请求文本、HTML、XML 或 JSON – 同时我们能够把这些外部数据直接载入网页的被选元素中。
-
jQuery Ajax本质就是 XMLHttpRequest,并对他进行了封装,方便调用!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| jQuery.ajax(...) 部分参数: url:请求地址 <---主要--- type:请求方式,GET、POST(1.9.0之后用method) headers:请求头 data:要发送的数据 <---主要--- contentType:即将发送信息至服务器的内容编码类型(默认: "application/x-www-form-urlencoded; charset=UTF-8") async:是否异步 timeout:设置请求超时时间(毫秒) beforeSend:发送请求前执行的函数(全局) complete:完成之后执行的回调函数(全局) success:成功之后执行的回调函数(全局) <---主要--- error:失败之后执行的回调函数(全局) <---主要--- accepts:通过请求头发送给服务器,告诉服务器当前客户端课接受的数据类型 dataType:将服务器端返回的数据转换成指定类型 "xml": 将服务器端返回的内容转换成xml格式 "text": 将服务器端返回的内容转换成普通文本格式 "html": 将服务器端返回的内容转换成普通文本格式,在插入DOM中时,如果包含JavaScript标签,则会尝试去执行。 "script": 尝试将返回值当作JavaScript去执行,然后再将服务器端返回的内容转换成普通文本格式 "json": 将服务器端返回的内容转换成相应的JavaScript对象 "jsonp": JSONP 格式使用 JSONP 形式调用函数时,如 "myurl?callback=?" jQuery 将自动替换 ? 为正确的函数名,以执行回调函数
|
案例一(使用SpringMVC)
- 编写一个AjaxController
1 2 3 4 5 6 7 8 9 10 11 12 13
| @RestController public class AjaxController {
@RequestMapping("/a1") public void ajax1(String name , HttpServletResponse response) throws IOException { if ("admin".equals(name)){ response.getWriter().print("true"); }else{ response.getWriter().print("false"); } }
}
|
- 导入jquery(在线的CDN与下载导入)
1 2
| <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script> <script src="${pageContext.request.contextPath}/statics/js/jquery-1.12.4.min.js"></script>
|
- 编写index.jsp测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Ajax测试</title> <%--<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>--%> <script src="${pageContext.request.contextPath}/statics/js/jquery-3.1.1.min.js"></script> <script> function a1(){ $.post({ url:"${pageContext.request.contextPath}/a1", data:{'name':$("#txtName").val()}, success:function (data,status) { alert(data); alert(status); } }); } </script> </head> <body> <%--onblur:失去焦点触发事件--%> 用户名:<input type="text" id="txtName" onblur="a1()"/> </body> </html>
|
- 设置静态资源不被拦截
1 2 3
| <mvc:resources mapping="/static/js/**" location="/js/"/>
|
6.3 数据返回案例
- 实体类User
1 2 3 4 5 6 7 8
| @Data @AllArgsConstructor @NoArgsConstructor public class User { private String name; private int age; private String sex; }
|
- Controller类
1 2 3 4 5 6 7 8
| @RequestMapping("/a2") public List<User> ajax2(){ List<User> list = new ArrayList<User>(); list.add(new User("admin",99,"男")); list.add(new User("boy",3,"男")); list.add(new User("girl",3,"女")); return list; }
|
- 前端界面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <input type="button" id="btn" value="获取数据"/> <table width="80%" align="center"> <tr> <td>姓名</td> <td>年龄</td> <td>性别</td> </tr> <tbody id="content"> </tbody> </table> <script src="${pageContext.request.contextPath}/statics/js/jquery-3.1.1.min.js"></script> <script> $(function () { $("#btn").click(function () { $.post("${pageContext.request.contextPath}/a2",function (data) { console.log(data) var html=""; for (var i = 0; i <data.length ; i++) { html+= "<tr>" + "<td>" + data[i].name + "</td>" + "<td>" + data[i].age + "</td>" + "<td>" + data[i].sex + "</td>" + "</tr>" } $("#content").html(html); }); }) }) </script> </body> </html>
|
6.4 注册提示案例
- Controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| @RequestMapping("/a3") public String ajax3(String name,String pwd){ String msg = ""; if (name!=null){ if ("admin".equals(name)){ msg = "OK"; }else { msg = "用户名输入错误"; } } if (pwd!=null){ if ("123456".equals(pwd)){ msg = "OK"; }else { msg = "密码输入有误"; } } return msg; }
|
- 前端界面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>ajax</title> <script src="${pageContext.request.contextPath}/statics/js/jquery-3.1.1.min.js"></script> <script>
function a1(){ $.post({ url:"${pageContext.request.contextPath}/a3", data:{'name':$("#name").val()}, success:function (data) { if (data.toString()=='OK'){ $("#userInfo").css("color","green"); }else { $("#userInfo").css("color","red"); } $("#userInfo").html(data); } }); } function a2(){ $.post({ url:"${pageContext.request.contextPath}/a3", data:{'pwd':$("#pwd").val()}, success:function (data) { if (data.toString()=='OK'){ $("#pwdInfo").css("color","green"); }else { $("#pwdInfo").css("color","red"); } $("#pwdInfo").html(data); } }); }
</script> </head> <body> <p> 用户名:<input type="text" id="name" onblur="a1()"/> <span id="userInfo"></span> </p> <p> 密码:<input type="text" id="pwd" onblur="a2()"/> <span id="pwdInfo"></span> </p> </body> </html>
|
7. 路径匹配
7.1 /**和/*区别
Wildcard |
Description |
? |
匹配任何单字符 |
* |
匹配0或者任意数量的字符 |
** |
匹配0或者更多的目录 |
Path |
Description |
/app/*.x |
匹配所有在app路径下的.x文件 |
/app/p?ttern |
匹配/app/pattern 和 /app/pXttern,但是不包括/app/pttern |
/**/example |
匹配 /app/example, /app/foo/example, 和 /example |
/app/**/dir/file. |
匹配/app/dir/file.jsp, /app/foo/dir/file.html,/app/foo/bar/dir/file.pdf, 和 /app/dir/file.java |
/**/*.jsp |
匹配任何的.jsp 文件 |
- /* : 匹配一级,即 /add , /query 等
- /** : 匹配多级,即 /add , /add/user, /add/user/user… 等
8. 文件操作
8.1 文件下载
- 准备:导入文件上传的jar包,commons-fileupload , Maven会自动帮我们导入他的依赖包 commons-io包;
1 2 3 4 5 6 7 8 9 10
| <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> </dependency>
<dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> </dependency>
|
- 配置bean(配置文件解析器):multipartResolver
【注意!!!这个bean的id必须为:multipartResolver , 否则上传文件会报400的错误!】
1 2 3 4 5 6 7 8
| <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <property name="defaultEncoding" value="utf-8"/> <property name="maxUploadSize" value="10485760"/> <property name="maxInMemorySize" value="40960"/> </bean>
|
CommonsMultipartFile 的 常用方法:
- String getOriginalFilename():获取上传文件的原名
- InputStream getInputStream():获取文件流
- void transferTo(File dest):将上传文件保存到一个目录文件中
- 下载步骤:
- 设置 response 响应头
- 读取文件 – InputStream
- 写出文件 – OutputStream
- 执行操作
- 关闭流 (先开后关)
- 代码实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| @RequestMapping(value="/download") public String downloads(HttpServletResponse response ,HttpServletRequest request) throws Exception{ String path = request.getServletContext().getRealPath("/upload"); String fileName = "基础语法.jpg";
response.reset(); response.setCharacterEncoding("UTF-8"); response.setContentType("multipart/form-data"); response.setHeader("Content-Disposition", "attachment;fileName="+URLEncoder.encode(fileName, "UTF-8"));
File file = new File(path,fileName); InputStream input=new FileInputStream(file); OutputStream out = response.getOutputStream();
byte[] buff =new byte[1024]; int index=0; while((index= input.read(buff))!= -1){ out.write(buff, 0, index); out.flush(); } out.close(); input.close(); return null; }
|
1
| <a href="/download">点击下载</a>
|
8.2 文件上传
- 导入相关依赖(见8.1.1)
- 配置文件解析器:multipartResolver(见8.1.2)
- 代码实现:
- 提交的表单,form标签method属性值必须为post,enctype属性值必须为multipart/form-data。
1 2 3 4 5
| <h3>SpringMVC文件上传</h3> <form action="fileUpload_2" method="post" enctype="multipart/form-data"> 选择文件:<input type="file" name="upload"> <input type="submit" value="上传"> </form>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| @RequestMapping("/fileUpload_2") public String fileUpload_2(HttpServletRequest request, MultipartFile upload) throws Exception { System.out.println("SpringMVC文件上传。。。");
String path = request.getSession().getServletContext().getRealPath("/upload/"); System.out.println(path); File file = new File(path); if (!file.exists()) { file.mkdirs(); }
String filename = upload.getOriginalFilename(); String uuid = UUID.randomUUID().toString().replace("-", ""); filename = uuid + "-" + filename; upload.transferTo(new File(path, filename)); return "success"; }
|
8.3 跨服务器上传文件
-
在8.2的环境下进行以下操作: 导入依赖,配置文件解析器
-
导入相关依赖:
1 2 3 4 5 6 7 8 9 10
| <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-core</artifactId> <version>1.18.1</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-client</artifactId> <version>1.18.1</version> </dependency>
|
- 前端界面:
1 2 3 4 5
| <h3>跨服务器文件上传</h3> <form action="fileUpload_3" method="post" enctype="multipart/form-data"> 选择文件:<input type="file" name="upload"> <input type="submit" value="上传"> </form>
|
- 控制器代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| @RequestMapping("/fileUpload_3") public String fileUpload_3(MultipartFile upload) throws Exception { System.out.println("SpringMVC文件上传。。。"); String path = "http://localhost:9090/uploads/"; String filename = upload.getOriginalFilename(); String uuid = UUID.randomUUID().toString().replace("-", ""); filename = uuid + "-" + filename; Client client = Client.create(); WebResource webResource = client.resource(path + filename); webResource.put(upload.getBytes()); return "success"; }
|
- 注意事项:
- 在本地测试时,在一个项目下创建两个模组、需要配置两个服务器(应用服务器和文件服务器),记得将端口号设置为不同。
- 在文件服务器的模组上新建uploads文件夹,否则上传时会报错(409异常)。
9. 异常处理
9.1 自定义异常处理流程
- 编写自定义异常类(作提示信息)
- 编写自定义异常处理器(处理器类实现HandlerExceptionResolver接口)
- 配置异常处理器(跳转到提示界面)
9.2 代码实现
- 控制器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Controller @RequestMapping("/user") public class usercontroller { @RequestMapping("/testException") public String testException() throws SysException{ System.out.println("testException执行了。。。"); try { int a = 10/0; } catch (Exception e) { e.printStackTrace(); throw new SysException("查询用户出现异常。。。"); } return "success"; } }
|
- 自定义异常类
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class SysException extends Exception{ private String message; public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public SysException(String message) { this.message = message; } }
|
- 自定义异常处理器(处理器类实现HandlerExceptionResolver接口)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class SysExceptionResolver implements HandlerExceptionResolver { public ModelAndView resolveException(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Object o, Exception ex) { SysException e = null; if(ex instanceof SysException){ e = (SysException) ex; }else { e = new SysException("系统正在维护。。。"); } ModelAndView mv = new ModelAndView(); mv.addObject("errorMsg",e.getMessage()); mv.setViewName("error"); return mv; } }
|
- 配置异常处理器
1 2
| <bean id="sysExceptionResolver" class="com.yang.exception.SysExceptionResolver"/>
|
- 其他界面
1 2 3 4 5 6 7 8
| <body> <h3>异常处理</h3> <a href="user/testException">异常处理</a> </body> /***************************************************************************************/ <body> ${errorMsg} </body>
|
10. 拦截器
拦截器 |
过滤器 |
它是SpringMVC框架自己的,只有使用了SpringMVC的工程才可以使用 |
它是Servlet规范中的一部分,任何Java Web工程都可以使用 |
只会拦截访问的控制器方法,如果访问的是jsp、html、css、image或者js是不会进行拦截的 |
在url-pattern中配置了/*之后,可以对所有要访问的资源过滤 |
- 控制器
1 2 3 4 5 6 7 8 9
| @Controller @RequestMapping("/user") public class UserController { @RequestMapping("/testInterceptor") public String testInterceptor(){ System.out.println("testInterceptor执行了。。。"); return "success"; } }
|
- 拦截器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class MyInterceptor_1 implements HandlerInterceptor {
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("MyInterceptor_1执行了。。。前1111"); request.getSession().invalidate(); request.getSession().removeAttribute(""); return true; }
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out.println("MyInterceptor_1执行了。。。后1111"); }
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { System.out.println("MyInterceptor_1执行了。。。最后1111"); } }
|
- 配置拦截器
1 2 3 4 5 6 7 8 9 10
| <mvc:interceptors> <mvc:interceptor> <mvc:mapping path="/user/*"/> <bean class="com.yang.interceptor.MyInterceptor_1"/> </mvc:interceptor> </mvc:interceptors>
|
- 运行结果
1 2 3 4 5
| MyInterceptor_1执行了。。。前1111 testInterceptor执行了。。。 MyInterceptor_1执行了。。。后1111 success.jsp执行了。。。 MyInterceptor_1执行了。。。最后1111
|
- 如果存在两个拦截器(且对同一个控制器进行拦截),则运行结果如下:
1 2 3 4 5 6 7 8
| MyInterceptor_1执行了。。。前1111 MyInterceptor_2执行了。。。前2222 testInterceptor执行了。。。 MyInterceptor_2执行了。。。后2222 MyInterceptor_1执行了。。。后1111 success.jsp执行了。。。 MyInterceptor_2执行了。。。最后2222 MyInterceptor_1执行了。。。最后1111
|
11. 相关注解
11.1 RequestParam
1 2 3 4 5 6 7 8 9
| @RequestMapping("/useRequestParam") public String useRequestParam(@RequestParam("name")String username, @RequestParam(value="age",required=false)Integer age){ System.out.println(username+","+age); return "success"; }
<a href="springmvc/useRequestParam?name=test">requestParam 注解</a>
|
11.2 RequestBody
11.3 PathVaribale
1 2 3 4 5 6 7 8 9
| @RequestMapping("/usePathVariable/{id}") public String usePathVariable(@PathVariable("id") Integer id){ System.out.println(id); return "success"; }
<a href="springmvc/usePathVariable/100">pathVariable 注解</a>
|
-
作用: 用于获取请求消息头。
-
属性:
- value:提供消息头名称
- required:是否必须有此消息头
-
一般不常用。
11.5 CookieValue
11.6 ModelAttribute
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @ModelAttribute public void showModel(User user) { System.out.println("执行了 showModel 方法"+user.getUsername()); } @RequestMapping("/testModelAttribute") public String testModelAttribute(User user) { System.out.println("执行了控制器的方法"+user.getUsername()); return "success"; }
<a href="springmvc/testModelAttribute?username=test">测试 modelattribute</a> 运行结果: 执行了showModel方法test 执行了控制器的方法test
|
11.7 SessionAttributes
-
作用:用于多次执行控制器方法间的参数共享。 只能作用于类上。
-
属性:
- value:用于指定存入的属性名称
- type:用于指定存入的数据类型。
-
通过@SessionAttributes注解设置的参数有3类用法:
- 在视图中通过request.getAttribute或session.getAttribute获取
- 在后面请求返回的视图中通过session.getAttribute或者从model中获取
- 自动将参数设置到后面请求所对应处理器的Model类型参数或者有@ModelAttribute注释的参数里面。
-
将一个参数设置到SessionAttributes中需要满足两个条件:
- 在@SessionAttributes注解中设置了参数的名字或者类型
- 在处理器中将参数设置到了model中
-
@SessionAttributes用户后可以调用SessionStatus.setComplete来清除,这个方法只是清除SessionAttribute里的参数,而不会应用Session中的参数。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
@RequestMapping("/testSessionAttributes") public String testSessionAttributes(Model model){ System.out.println("testSessionAttributes..."); model.addAttribute("msg","成功!"); return "success"; } @RequestMapping("/getSessionAttributes") public String getSessionAttributes(ModelMap modelMap){ System.out.println("getSessionAttributes..."); String msg = (String)modelMap.get("msg"); System.out.println(msg); return "success"; } @RequestMapping("/delSessionAttributes") public String delSessionAttributes(SessionStatus status){ System.out.println("delSessionAttributes..."); status.setComplete(); return "success"; }
|
WebClient了解、使用!